Asteroids
This page will lead you through the steps necessary for programming an Asteroids clone in Logo
You will end up making four separate programs in the editor. One of which controls all the others. You should save the whole Logo workspace so that you can reload your programs later.
This program draws a very basic asteroid.
First of all though it draws a white asteroid where the asteroid was last turn.
It then draws a black asteroid at the asteroid's new position.
to drawRock :oldX :oldY :newX :newY :angle
rt :angle
if (AND (:newX > -300) (:newX < 300) (:newY > -300) (:newY < 300)) [
setpencolor (list 255 255 255)
pu setxy :oldX :oldY pd
fd 10 rt 45 fd 12 rt 70 fd 5 rt 80 fd 11 rt 50 fd 10 rt 115 fd 5
pu setxy :newX :newY pd
setpencolor (list 0 0 0)
fd 10 rt 45 fd 12 rt 70 fd 5 rt 80 fd 11 rt 50 fd 10 rt 115 fd 5
]
lt :angle
end
Test the program by typing drawRock 100 100 100 100 100 into the command line.
| 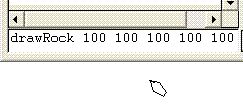
The turtle is rotated to the :angle value for the current asteroid.
A four value AND function checks that the asteroid is still in the box.
If the asteroid is in the box then the previous drawing of the asteroid is erased and it is then redrawn in its new location.
Finally the turtle is lined up in the right direction
|
Now you need to make a lot of asteroids and then move them about on the screen.
This pogram makes :n asteroids.
to makeRock :n
make "xCo (array :n 0)
make "yCo (array :n 0)
make "xVe (array :n 0)
make "yVe (array :n 0)
make "th (array :n 0)
for [c 0 :n-1 1] [
setitem :c :xCo ((random 500)-250)
setitem :c :yCo ((random 500)-250)
setitem :c :xVe ((random 10)-5)
setitem :c :yVe ((random 10)-5)
setitem :c :th random 360
]
end
|
You can test this program by typing makeRock 10 but it won't do anything as it doesn't send an output to the screen!
If you type makeRock 10 and you get an error message then you have done something wrong!
:xCo & yCo are random start coordinates.
:xVe & :yVe are random start velocities.
These are loaded into arrays along with a random starting angle for the asteroid.
|
Now you need to be able to move the rocks about.
to moveRock :n
for [ c 0 :n-1 1] [
make "xCoOld item :c :xCo
setitem :c :xCo ((item :c :xCo)+(item :c :xVe))
make "yCoOld item :c :yCo
setitem :c :yCo ((item :c :yCo)+(item :c :yVe))
drawRock :xCoOld :yCoOld item :c :xCo item :c :yCo item :c :th
]
end
|
This is another piece of code that can't be tested independently.
|
So what you now need is the main program loop that calls and controls all the others.
to rocks
ht
make "cn 0
pu setxy -300 -300 pd
repeat 4 [fd 600 rt 90]
pu home pd
makeRock 10
while [:cn=0] [moveRock 10]
st
end
|
The program hides the turtle and then draws a big square box. The turtle is then returned to the origin.
After this, makeRock is called and told to make 10 rocks.
MoveRock is then called repeatedly until a variable called :cn != 0 (Doesn't equal 0). I haven't programmed :cn into the program yet so the while loop continues until you hit HALT.
|
|
|
|
|
Last updated 23rd February 2010