Built in commands |
Creating Lists
make "a (list 2 3 4 5)
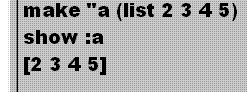
Copying Lists
make "a (list 2 3 4 5)
show :a
make "b :a
show :b
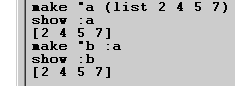
|
Joining Two Lists
To join two items use this:
make "a (list 2 3 4 5)
make "b (list 7 8 9 )
show :a
show :b
make "c sentence :a :b
show :c
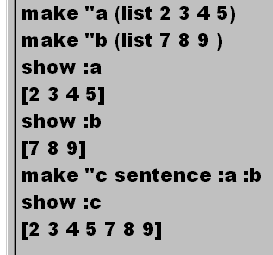
|
Joining Several Lists
To join three or more lists use this
make "a (list 2 3 4 5)
make "b (list 7 8 9 )
show :a
show :b
make "c (sentence :a :b :a)
show :c
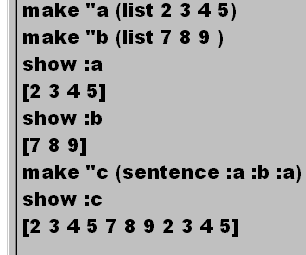
|
fput
Puts an item at the front of a list
make "a (list 2 3 4 5)
make "a fput 8 :a
show :a
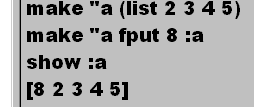
lput
Puts an item at the end of a list
make "a (list 2 3 4 5)
make "a lput 8 :a
show :a
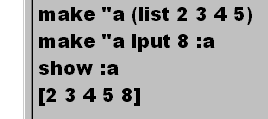
|
first
Copies an item from the front of a list leaving the list unchanged.
make "a (list 2 4 5 7)
show :a
make "b first :a
show :b
show :a
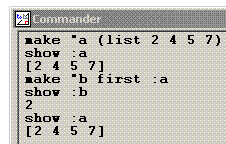
butfirst
Copies all except the first item of a list leaving the list unchanged.
make "a (list 2 4 5 7)
make "b butfirst :a
show :b
show :a
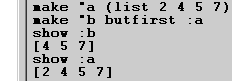
|
|
last
To copy the last member of a list use:
make "a (list 2 3 4 5)
show last :a
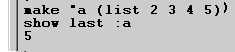
|
butlast
To copy all but the last member of a list use:
make "a (list 2 3 4 5)
make "b butlast :a
show :b
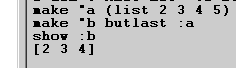
|
reverse
Reverses a list:
make "a (list 2 3 5 7)
make "b reverse :a
show :b
show :a
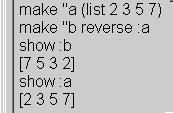
|
Logic Operations |
memberp
Returns true iff the search element is a member of the list.
make "a (list 2 3 5 7)
show memberp 9 :a
show memberp 2 :a
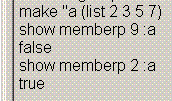
|
equalp
Returns true iff the two lists contain the same items in the same order.
make "a (list 2 3 5 7)
make "b (list 7 5 3 2)
make "c (list 2 3 5 7)
show equalp :a :c
show equalp :b :c
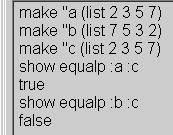
|
emptyp
Returns true iff the list is empty
make "a (list 2 3 5 7)
make "b []
show emptyp :a
show emptyp :b
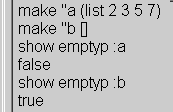
|
lessp
Returns true iff the first element is less than the second.
make "a 2
make "b 5
show lessp :a :b
show lessp :b :a
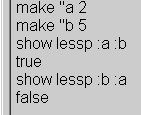
|
greaterp
Returns true iff the first element is greater than the second.
make "a 2
make "b 5
show greaterp :a :b
show greaterp :b :a
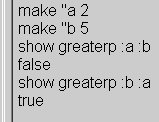
|
|
Useful Procedures
Download the logolists.lgo file
|
elementn
Returns the nth element of a list.
to elementn :list :element
repeat :element-1 [make "list butfirst :list]
output first :list
end
Repeat repeats the code in the bracket the number of times set by the value before the brackets. Output sends the value calculated by the procedure to the code which called it.
Call and test the procedure as follows:
make "a (list 2 3 4 5 7)
show elementn :a 2
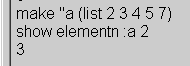
|
removen
Removes the item at position 'element' from the list and returns the modified list.
to removen :list :element
localmake "newlist []
repeat count :list [
ifelse :element=repcount [][
make "newlist lput (elementn :list repcount) :newlist ]]
output :newlist
end
Localmake ensures that operations are only carried out on a local variable. This prevents alterations to any variables outside the local procedure.
Count returns the number of items in the list.
Ifelse executes the first code block if its condition is true or the second if it is false.
Execute and test the procedure as follows:
make "a (list 2 3 4 5 7)
show :a
make "a removen :a 3
show :a
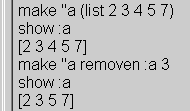
|
put
Places list2 into list1 after the nth item in list1
to put :list1 :n :list2
localmake "newlist []
repeat :n [
make "newlist lput (first :list1) :newlist
make "list1 butfirst :list1 ]
make "newlist (sentence :newlist :list2 :list1)
output :newlist
end
Call and test using:
make "a (list 2 3 4 5 7)
make "b (list 8 9)
make "c put :a 2 :b
show :c
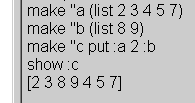
|
swap
Swaps element n for element m
to swap :list :n :m
make "nthelement elementn :list :n
make "mthelement elementn :list :m
ifelse (:n>:m) [ make "list removen :list :n
make "list removen :list :m] [
make "list removen :list :m
make "list removen :list :n]
ifelse (:n>:m) [ make "list put :list :n-2 :mthelement
make "list put :list :m-1 :nthelement] [
make "list put :list :m-2 :nthelement
make "list put :list :n-1 :mthelement]
output :list
end
Call and test using:
make "a (list 1 2 3 4 5 6 7 8 9)
show swap :a 1 9
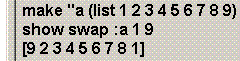
|
Set Operations
Download the logolists.lgo file
|
union
Joins two lists/sets omitting repeated items.
to union :la :lb
make "c []
repeat count :la [
ifelse (memberp first :la :c) [][
make "c sentence first :la :c ]
make "la butfirst :la ]
repeat count :lb [
ifelse (memberp first :lb :c) [][
make "c sentence first :lb :c ]
make "lb butfirst :lb ]
output :c
end
Call and test using:
make "a (list 1 2 3 4 3 5 6)
make "b (list 5 9 9 3 8 7 11 21)
show :a
show :b
show union :a :b
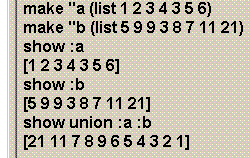
|
intersection
Overlap of two lists/sets omitting repeated items.
to intersection :la :lb
make "c []
repeat count :la [
if (memberp first :la :lb) [
ifelse (memberp first :la :c) [][
make "c sentence first :la :c]
]
make "la butfirst :la ]
output :c
end
Call and test using:
make "a (list 1 2 3 4)
make "b (list 3 4 5 6)
show intersection :a :b
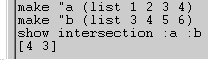
|
complement
Lists non-overlapping elements of two lists/sets omitting repeated items.
to complement :la :lb
make "c []
make "la2 :la
repeat count :la [
ifelse (memberp first :la :lb) [][
ifelse (memberp first :la :c) [][
make "c sentence first :la :c]]
make "la butfirst :la ]
repeat count :lb [
ifelse (memberp first :lb :la2) [][
ifelse (memberp first :lb :c) [][
make "c sentence first :lb :c]]
make "lb butfirst :lb ]
output :c
end
Call and test using:
make "a (list 1 2 3 4)
make "b (list 3 4 5 5 6 7)
show complement :a :b
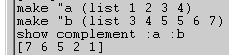
|
Last updated 27th May 2010