Constructing a LOGO implementation of 'Pong'.
Pong is a classic arcade game. You have to bounce the puck off the walls etc.
The following steps are necessary to achieve a simple working version of the game
- Read the keyboard to get input from the player
- Draw and move the bat.
- Draw the boundary box.
- Draw and move the puck.
- Handle collisions with the sides and the bat.
- Start and finish the game
Work through each stage in turn so that you can build up your Pong program.
Reading the keyboard |
Direct via the Command line.
Type or paste these two lines of code into the command line.
keyboardon [print keyboardvalue]
setfocus [MSWLogo screen]
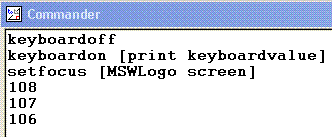
This will now print the numerical value of most of the keys that you press. Note the values of the keys that you want to use to control a game.
To turn the keyboard off type this:
keyboardoff
|
A Keyboard Reading Procedure
This procedure returns the value of the last key pressed.
to keyread
keyboardon [make "keyval keyboardvalue]
setfocus [MSWLogo screen]
output :keyval
keyboardoff
setfocus [MSWLogo commander]
end
Test it with this command line code:
repeat 30 [print keyread wait 60]
|
Drawing and moving the bat. Drawing the box. |
Drawing a bat.
Procedure to draw a bat.
to drawbat :xpos
setfc [255 255 255]
pu setxy -200 -215 pd
bitblock 400 10
setfc [0 0 0]
pu setxy :xpos -215 pd
bitblock 40 10
end
Test with the command line code:
cs ht drawbat 100
|
setfc sets the fill colour.
setxy positions the turtle at point :x :y
bitblock shades in a rectangle
Do this in white to clear the previous paddle and then black to draw it at its new position.
|
Moving the bat.
to movebat
make "retval keyread
if :retval>0 [
if :retval=97 [
make "batspeed :batspeed-1 ]
if :retval=115 [
make "batspeed :batspeed+1 ]
if :retval=120 [
make "batspeed 0 ]]
make "xInd :xInd+:batspeed
if :xInd>160 [make "xInd 160
make "batspeed 0 ]
if :xInd<-200 [make "xInd -200
make "batspeed 0 ]
pu setxy :xInd -180 pd
drawbat :xInd
end
You need a separate procedure to test this code.
|
Test using this procedure. (a= left, s=right and x=stop) The test ends after 100 cycles of the 'movebat' procedure.
to testmovebat
make "xInd 0
make "batspeed 0
make "retval 0
repeat 100 [
movebat
wait 2
]
end
Start the test with:
testmovebat
The keyread procedure is used to interogate the keyboard. xInd stores the bat's position and batspeed stores its speed. These are allowed to vary within the maximum positions which will be set by the box which will contain the game.
|
Drawing the box
This procedure draws the box. Test by using box
to box
cs
setpensize [5 5]
pu setxy -210 -210 pd
repeat 3 [ fd 420 rt 90]
end
|
|
Drawing and moving the puck
|
This is the longest and most complicated part of the program.
to movepuck
make "stopflag 0
make "x random 100
make "y random 100
make "xvel 3+random 2
make "yvel 3+random 2
pu setxy :x :y pd
setpc (list 0 0 0)
circle 4
do.until[
pu setxy :x :y pd
setpc (list 255 255 255)
circle 4
movebat
make "x :x+:xvel
make "y :y+:yvel
if :x>200 [make "x 200
make "xvel :xvel*(-1)]
if :x<-200 [make "x -200
make "xvel :xvel*(-1)]
if :y>200 [make "y 200
make "yvel :yvel*(-1)]
if :y<-220 [make "stopflag 1]
if :y<-200 [
ifelse :x>:xInd [
ifelse :x<:xInd+40 [
make "yvel :yvel*-1
make "xvel :xvel+:batspeed
make "y -200][make "stopflag 1]][make "stopflag 1] ]
pu setxy :x :y pd
setpc (list 0 0 0)
circle 4
wait 2
][:stopflag = 1]
end
|
Test by using: movepuck
stoplflag starts at 0 and causes the game to finish if it is changed to 1.
The puck starts at a random position with a random velocity.
The puck is drawn in black (RGB 0 0 0 ) using the circle command and erased by redrawing it at the same location in white (RGB 255 255 255).
The do.until loop controls the main part of the game. It exits when stoplflag =1 this happens when the puck escapes from the bottom of the box.
The puck moves independently in the x and y directions. If it reaches the edges of the box then its velocity is multiplied by -1 to reverse its direction. If the puck reaches the bottom of the box in the same place as the bat then it bounces back up otherwise the game ends.
The speed of the bat affects the angle with which the puck bounces off the bat.
Start Game
A small procedure is needed to set the game running.
to startgame
make "xInd 0
make "retval 0
make "batspeed 0
cs ht
box
movepuck
end
Start the game with startgame
Extensions
The most obvious thing to do is to make this a two player game.
To do this you would need to remove the top edge of the box. Add a second bat with its own set of keys and control software.
Alter the escape conditions in the main program.
It would be best to save the single player version under a new name so that you don't destroy it!
Have fun!!
LOGOPong Full program listing.
|
|
|
|
|
Last modified 6th February 2012